What Is a Function?
Functions are the essential building blocks of your scripts. They are used to combine multiple statements in one place.
A function can return a result, which can then be assigned to a variable.
Functions can have one or more arguments, or no arguments at all. Arguments are listed in parentheses after the name of the function. There are even functions for which the number of arguments depends on how you are using the function.
JavaScript supports two kinds of functions: those that are built into the language, and those you create yourself.
Special Built-in Functions
The JavaScript language includes several built-in functions. Some of them let you handle expressions and special characters, and convert strings to numeric values.
For example, escape() and unescape() are used to convert characters that have special meanings in HTML code, characters that you cannot just put directly into text. For example, the angle brackets, "<" and ">", delineate HTML tags.
The escape function takes as its argument any of these special characters, and returns the escape code for the character. Each escape code consists of a percent sign (%) followed by a two-digit number. The unescape function is the exact inverse. It takes as its argument a string consisting of a percent sign and a two-digit number, and returns a character.
Another useful built-in function is eval(), which evaluates any valid mathematical expression that is presented in string form. The eval() function takes one argument, the expression to be evaluated.
anExpression = "6 * (9 % 7)"; total = eval(anExpression); // Assigns the value 12 to the variable total.
document.write(anExpression + " is " + total); |
To run the code, paste it into JavaScript Editor, and click the Execute button.
Consult the language reference for more information about eval() and other built-in functions.
Creating Your Own Functions
You can create your own functions and use them where you need them.
Example:
The Math object has a method called random(), which returns a random number between 0 and 1. Our new function, getRandom (min, max), uses Math.random() to calculate and return a random integer number between the minimum and maximum values.
function getRandom(min, max)
{
var randomNum = Math.random() * (max-min);
// Round to the closest integer and return it
return(Math.round(randomNum) + min);
}
|
To test it, write a for loop to call the getRandom() function 100 times. This time, you are going to use JavaScript Editor's Code Templates to write the loop for you.
Code templates are reusable chunks of code which can greatly increase your speed of coding.
|
To insert a code template into your document, just type in the first few letters of the template and press Ctrl+Space (or just press Ctrl+Space when your cursor is on a blank line to see the list of all available templates). In this case, type the letter f and press Ctrl+Space. The list of all matching templates pops up, allowing you to select the one you need. Select the for template:
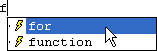
Sometimes it is difficult to remember which template you need if you have lots of them. In this case, select Insert/Code Template from the menu (or press Ctrl+T) to open the Code Template dialog. Type in the first few letters, or double-click the name of the template to insert it into your document.
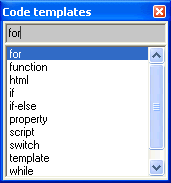
Each code template can have one or more properties, which you can fill-in before inserting the template into your document. For example, type for followed by Ctrl+Space, and the following property dialog pops up:
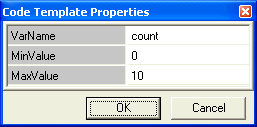
Change the MaxValue from 10 to 100 and click OK:
for (count = 0; count < 100; count++)
{
}
Complete the function by adding a call to getRandom():
function getRandom(min, max)
{
var randomNum = Math.random() * (max-min);
// Round to the closest integer and return it
return(Math.round(randomNum) + min);
}
// Call the function 100 times to test it
for(count=0; count<100; count++)
{
document.write(getRandom(1, 6));
} |
To run the code, paste it into JavaScript Editor, and click the Execute button.
Exercise: Create a function that simulates the roll of the dice. Tip: since the dice can only have values between 1 and 6, our rollDice() function will call the getRandom() above and pass 1 and 6 as the minimum and maximum values.
Next
|